Vercel Edge Config
Overview
By leveraging Vercel Edge Functions, you can easily and performantly deliver personalized content to your website's visitors. These functions are deployed globally via Vercel's Edge Network and allow you to move server-side logic to the Edge, giving your visitors faster access. With NextJS applications on Vercel, you can experiment at the edge using Statsig's feature flags and experimentation capabilities. The Statsig Vercel integration lets you easily bootstrap your Vercel projects by directly pushing Statsig Configs to Vercel's Edge Config.
Configuration
Go to the Vercel Marketplace to install the Statsig app for Vercel. In the setup, you'll be able to map Statsig projects <-> Edge Configs, and Statsig will keep project configs synced with the specified Edge Config.
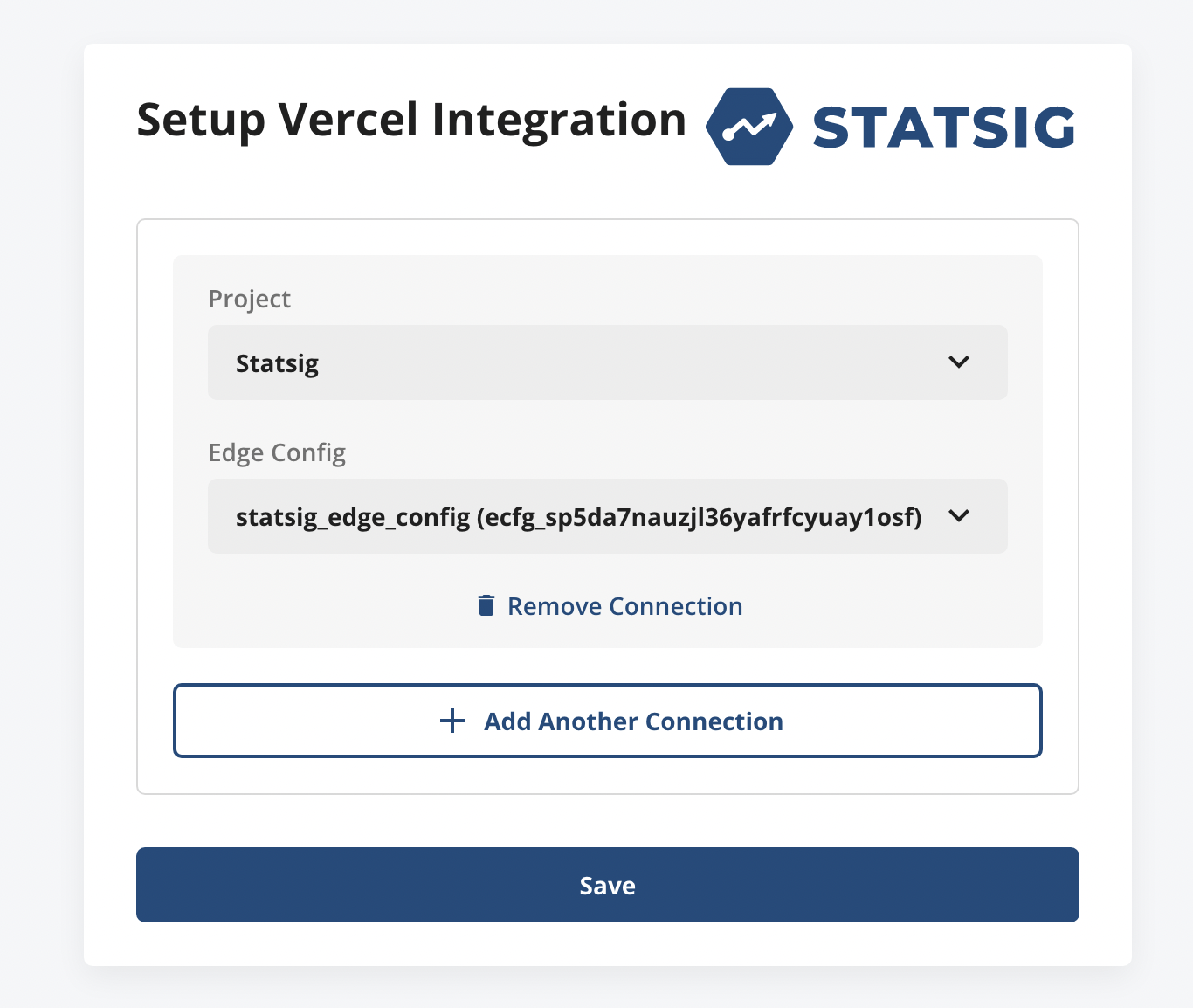
After setting up the mapping, you'll be given an Edge Config Connection String, which should be saved to the EDGE_CONFIG environment variable in your Vercel project. You can read your Statsig configs from the Edge Config Item Key provided. See here for a sample project.
After setting up the configuration, you can go to Project Settings -> Integrations and click on the Vercel Card to go to an integration configuration page for the project.
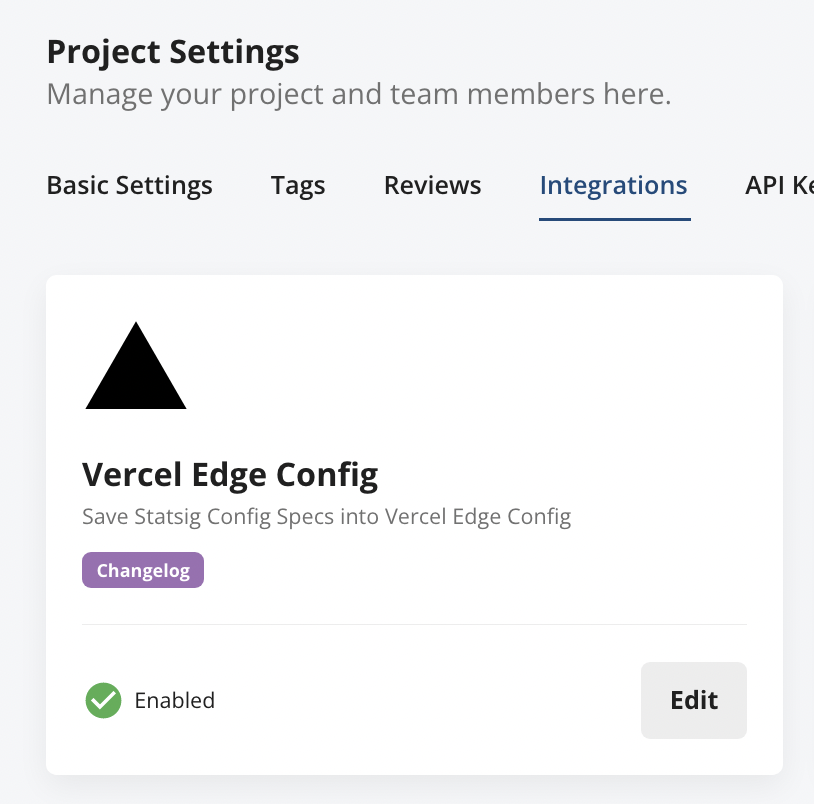
Known Limitations
Vercel Edge Config has maximum size limits that may prevent Statsig from pushing configs into your Edge Config. See here for the latest Vercel Edge Config limits.
Statsig ID Lists are not currently synced into the Edge Config.